EventEmitter
Evented JavaScript for the browser
I'd like to have something like this work:
var Events=require('events'),
test=new Events.EventEmitter,
scope={
prop:true
};
test.on('event',function() {
console.log(this.prop===true);//would log true
});
test.emit.call(scope,'event');
But, unfortunately, the listener doesn't even get called. Is there any way to do this w/ EventEmitter? I could Function.bind
to the listener, but, I'm really hoping EventEmitter
has some special (or obvious ;) way to do this...
Thanks for the help!
Source: (StackOverflow)
How can I debug my application witch throw this error:
(node) warning: possible EventEmitter memory leak detected. 11 listeners added. Use emitter.setMaxListeners() to increase limit.
Trace
at Socket.EventEmitter.addListener (events.js:160:15)
at Socket.Readable.on (_stream_readable.js:653:33)
at Socket.EventEmitter.once (events.js:179:8)
at TCP.onread (net.js:527:26)
I could not find the assumed leaking object for increasing listener limit by .setMaxListeners(0);
SOLUTION (from fardjad and jan salawa)
With jan salawa's searches I found a working library (longjohn) for increasing stack traces verbose. With fardjad's response I have found that we have to prototype EventEmitter.addListener
AND EventEmitter.on
.
With this solution I could get this new trace :
(node) warning: possible EventEmitter memory leak detected. 11 listeners added. Use emitter.setMaxListeners() to increase limit.
Trace
at EventEmitter.addListener.EventEmitter.on (xxx/main.js:44:15)
at Readable.on (_stream_readable.js:653:33)
at ServerResponse.assignSocket (http.js:1072:10)
at parser.onIncoming (http.js:1979:11)
at parserOnHeadersComplete (http.js:119:23)
at socket.ondata (http.js:1912:22)
at TCP.onread (net.js:510:27)
Source: (StackOverflow)
I am getting following warning:
(node) warning: possible EventEmitter memory leak detected. 11 listeners added. Use emitter.setMaxListeners() to increase limit.
Trace:
at EventEmitter.<anonymous> (events.js:139:15)
at EventEmitter.<anonymous> (node.js:385:29)
at Server.<anonymous> (server.js:20:17)
at Server.emit (events.js:70:17)
at HTTPParser.onIncoming (http.js:1514:12)
at HTTPParser.onHeadersComplete (http.js:102:31)
at Socket.ondata (http.js:1410:22)
at TCP.onread (net.js:354:27)
I wrote code like this in server.js:
http.createServer(
function (req, res) { ... }).listen(3013);
How to fix this ?
Source: (StackOverflow)
I am at the end of my wits, nerves, everything, for the past 4 hours I am TRYING to debug and reading all over the internet what the problem might be with this.
So, I am working on mocking up a mobile application and my logic is like this:
- If the user has logged in the past, we have data in localStorage about that, then show him the listing. this is offline
- If we are connected to the internet, show the listing first and in the background check to see if he is still logged in
- If HE ISN'T, redirect to login page.
On the main Home view, I am evaluating the above statements, first I show him the listing if he has localStorage data, then I check online and redirect to login if necessary.
Whenever I have two $state or $locations it loops. Like the browser almost freezes, lucky it's Chrome. and I get the Cannot call method 'insertBefore' of null
. From what I read, a known angular.ui router issue, if used bad or some other mystical problem.
Some Code:
These event emitters and receivers, which I call in the return of my StateManagement Services
//Notification Helpers, also exposed, to keep them in the same scope tree.
function emitNotification (event, data) {
console.log('Emitting event: ' + event);
$rootScope.$emit(event, data);
}
function emitListen (event, fn) {
console.log('Listening for: ' + event);
$rootScope.$on(event, function (e, data) {
fn(data);
});
}
.
.
.LOGIC
//Update the userData in localStorage and in .value
$auth.storage.set('userData', data);
userData = $auth.storage.get('userData'); //TODO: Redundant, store it directly, is it ok?
emitNotification('event:auth-okServer', data);
//return 'ok-server';
... return my service
},
loginSuccess: function(data){
emitNotification('event:auth-loginSuccess', data);
},
loginFailed: function(data){
emitNotification('event:auth-loginFailed', data);
},
notify: emitNotification,
listen: emitListen
And this is in my controller:
.config(function config($stateProvider) {
$stateProvider
.state('sidemenu.home', {
url: '/home',
views: {
'topView': {
controller: 'HomeCtrl',
templateUrl: 'home/home.tpl.html'
}
}
})
.state('sidemenu.home.list', {
url: '/list',
templateUrl: 'home/home-list.tpl.html',
controller: 'ListCtrl'
})
.state('sidemenu.home.login', {
url: '/login',
templateUrl: 'home/home-login.tpl.html',
controller: 'LoginCtrl'
})
.state('sidemenu.home.register', {
url: '/register',
templateUrl: 'home/home-register.tpl.html',
controller: 'RegisterCtrl'
})
.state('sidemenu.home.coach', {
url: '/coach',
templateUrl: 'home/home-coach.tpl.html',
controller: 'CoachCtrl'
})
;
})
.controller('HomeCtrl', function HomeController($scope, $state, $location, localState) {
/**
* EVENT EMITTERS
* @event:auth-loginSuccess - Login has been succesfull, let everybody know.
* @event:auth-loginFailed - Login has failed, do something.
* @event:auth-okServer - Server challenge ok, user is logged in.
* @event:auth-okLocal - Local challenge ok, user appears to be logged in.
* @event:auth-login - Go to login
* @event:general-coach - Start the coach
**/
/**
* ===== FIRST LOGIC =====
**/
//We are placing emitters in the same children-parent tree scope, the one from localState, and we're listening to them.
localState.listen('event:auth-okLocal', function(data) {
console.log('EVENT: auth-okLocal has been triggered');
//$state.transitionTo('sidemenu.home.list');
$location.path('/sidemenu/home/list');
//First we will be redirected on listpage
});
localState.listen('event:auth-login', function() {
console.log('EVENT: auth-login has been triggered');
$location.path('/sidemenu/home/login');
});
//Server check fails, redirect to login
localState.listen('event:general-coach', function(data) {
console.log('EVENT: general-coach has been triggered');
//$state.transitionTo('sidemenu.home.coach');
$location.path('/sidemenu/home//coach');
});
//After we have all the listeners in place do the checking & broadcasting.
localState.check();
What am I doing wrong?
I have debugged the emitters, my check() function which creates all the emitters, I have tried several combinations on ui.router and I have miserably failed :).
Source: (StackOverflow)
I can't figure out why I can't make my server to run emit function.
Here's my code:
myServer.prototype = new events.EventEmitter;
function myServer(map, port, server) {
...
this.start = function () {
console.log("here");
this.server.listen(port, function () {
console.log(counterLock);
console.log("here-2");
this.emit('start');
this.isStarted = true;
});
}
listener HERE...
}
The listener is:
this.on('start',function(){
console.log("wtf");
});
All the console types is this:
here
here-2
Any idea why it wont print 'wtf'
?
Source: (StackOverflow)
Lets say I have 3 exposed functions: user, posts, articles
all of which needs to emit messages to a file called mediator where all of the events are set.
Currently I'm have trouble doing so
In the mediator file i have something like so:
var EventEmitter = require('events').EventEmitter;
, pubsub = new EventEmitter();
exports.pubsub = new EventEmitter()
pubsub.on('loggedIn', function(msg) {
console.log(msg);
});
and in the user, post and article functions. Something like so:
var mediator = require('../config/mediator')
, _ = require('underscore')
exports.account = function(req, res) {
var returned = _.omit(req.user._doc, 'password' )
mediator.pubsub.emit('loggedIn', 'A User logged in');
res.send(returned);
};
The emit is getting completely ignored, no error or anything. Not sure if I'm doing it right so any direction would be appreciated. The desired return is work as expected though.
Source: (StackOverflow)
I want to emit events from one file/module/script and listen to them in another file/module/script. How can I share the emitter variable between them without polluting the global namespace?
Thanks!
Source: (StackOverflow)
I see this pattern in quite a few Node.js libraries:
Master.prototype.__proto__ = EventEmitter.prototype;
(source here)
Can someone please explain to me with an example, why this is such a common pattern and when it's handy?
Source: (StackOverflow)
I have and application written in NodeJS with Express and am attempting to use EventEmitter to create a kind of plugin architecture with plugins hooking into the main code by listening to emitted events.
My problem comes when a plugin function makes an async request (to get data from mongo in this case) this causes the plugin code to finish and return control back to the original emitter which will then complete execution, before the async request in the plugin code finishes.
E.g:
Main App:
// We want to modify the request object in the plugin
self.emit('plugin-listener', request);
Plugin:
// Plugin function listening to 'plugin-listener', 'request' is an arg
console.log(request);
// Call to DB (async)
this.getFromMongo(some_data, function(response){
// this may not get called until the plugin function has finished!
}
My reason for avoiding a callback function back to the main code from the 'getFromMongo' function is that there may be 0 or many plugins listening to the event. Ideally I want some way to wait for the DB stuff to finish before returning control to the main app
Many Thanks
Source: (StackOverflow)
I am trying to emit from one EventEmitter to another EventEmitter, or find an alternative solution. The code below doesn't function.
Multiple emitter example
var events = require('events');
var eventEmitterOne = new events.EventEmitter();
var eventEmitterTwo = new events.EventEmitter();
eventEmitterTwo.on('fireEvent', function() {
console.log('event fired');
});
eventEmitterOne.emit('fireEvent');
I'm looking to find out if the following is possible and whats changes I would need to make to the following code to make it functional. Or is there another way of emitting events across multiple emitters. Thanks for your time.
Source: (StackOverflow)
I have written a basic angular application that utilises the EventEmitter class, however i cannot get the listening component to catch the event.
Here is my code (using alpha.27 on Angular2 / TypeScript 1.5 compiling to ES5)
Apologises for the verbose example.
Any advice on what i am incorrectly doing what would be greatly appreciated.
import {Component, View, EventEmitter} from 'angular2/angular2';
@Component({
selector: 'login',
events : ['loggedIn']
})
@View({
template: '<button type="button" (click)="submitForm()">Click Me</button>'
})
export class Login {
loggedIn = new EventEmitter();
constructor() {
}
submitForm() {
console.log("event fired");
this.loggedIn.next({});
}
}
@Component({
selector: 'app'
})
@View({
template: "<div>This is the application</div>"
})
export class App {
constructor() {
}
}
@Component({
selector: 'root'
})
@View({
template: '<app [hidden]=!showApp></app><login (loggedIn)="loggedIn()" [hidden]=showApp></login>',
directives: [ App, Login ]
})
export class Root {
showApp:boolean;
constructor() {
this.showApp = false;
}
loggedIn() {
console.log("event caught");
this.showApp = true;
}
}
Source: (StackOverflow)
I was just introduced to node.js's EventEmitters and love the way the code is structured much more that callbacks, and even using libraries like async. I completely understand the basics when there is a simple workflow, i.e.:
this.register = function(email, pass, confirm) {
var newCustomer = {email:email, pass:pass, confirm: confirm};
this.emit("newRegistration", newCustomer);
};
var _validate = function(customer{
this.emit("validated", customer);
};
//...
this.on("newReigstration", _validate);
this.on("validated", _insert);
Makes perfect sense. My question is what to do with functions that are commonly called. For instance, I may have 50 endpoints where I am passed a customer ID, and I need to go and get the customer out of the db, something like this:
Customer.getCustomer(customerId, function(err, customer) {
// Got the customer, now go do something.
});
I can't just wire up an event to the act of retrieving the customer, because there are 50 different things to be done after "getting the customer". So I couldn't do something like this:
this.on("customerRetrieved", task1);
this.on("customerRetrieved", task2);
this.emit("customerRetrieved", customer); // In getCustomer
Because both would be called, and I only want to call one each time. The only thing I can think of is do something like this:
this.on("customerRetrievedForTask1", task1);
this.on("customerRetrievedForTask2", task2);
But that seems clunky, not to mention dangerous if I accidentally give it the same name as something that already exists. What is the convention for these commonly called functions?
Source of code example
Source: (StackOverflow)
In Node.js I was able to make a WordPress clone rather easy using the EventEmitter to replicate and build a hooks-system into the CMS core, which plugins could then attach to.
I now need this same level of extensibility and core isolation for my CMS written in and ported to Go. Basically I have the core finished now, but in order to make it truly flexible I have to be able to insert events (hooks) and to have plugins attach to these hooks with additional functionality.
I don't care about recompiling (dynamic / static linking), as long as you don't have to modify the core to load plugins - the CMS core should never be modified. (like WP, Drupal etc.)
I noticed there's a few rather unknown projects, trying to implement events in Go looking somewhat similar to EventEmitter in Node.js:
https://github.com/CHH/eventemitter
https://github.com/chuckpreslar/emission
Since those 2 projects above haven't gained much popularity and attention somehow I feel this way of thinking about events might now be how we should do it in Go? Does this mean Go is maybe not geared to this task? To make truly extendable applications through plugins?
Doesn't seem like Go has events built into its core, and RPC doesn't seem like a valid solution for integrating plugins into your core application as were they built in natively, and as if they were part of the main application itself.
What's the best way for seamless plugin integration into your core app, for unlimited extension points (in core) without manipulating core every time you need to hook up a new plugin?
Source: (StackOverflow)
I have code to log every connection to my HTTP-server on a socket level and also log any incoming data.
This code was originally written for NodeJS 0.8 and works good there.
No my project is migrated to 0.10.24 and socket logging code stopped working.
Here is my code:
var netLogStream = fs.createWriteStream('net.log');
(function(f) {
net.Server.prototype.listen = function(port) {
var rv = f.apply(this, arguments); // (1)
rv.on('connection', function(socket) { // (2)
socket.on('data', function(data) {
data.toString().split('\n').forEach(function(line) { // (3)
netLogStream.write('... some logging here ... ' + line);
});
});
});
return rv;
};
})(net.Server.prototype.listen);
On 0.10 I can get to (1)
and get Socket
instance on (2)
but I never get to (3)
. Same time my whole application works fine without any issues.
ADD: My server is created with Express@3.4.x
Source: (StackOverflow)
I'm building a reporting service, where one process submits a query, retrieves a token, then hands off the token to another process, from which we query the reporting service and stream the results back.
Given this code, how should I implement the blocking call until paused is false?
var util = require('util')
, events = require('events')
, pg = require('pg')
// QueryHandler is an EventEmitter
function QueryHandler(sql) {
this.paused = true
pg.connect(connectionString, function(err, client) {
// error handling ignored for sake of illustration
var query = client.query(sql)
query.on('row', function(row) {
if (this.paused) {
// Somehow block until paused === false
}
this.emit(row)
}.bind(this))
}.bind(this))
}
util.inherits(QueryHandler, events.EventEmitter)
QueryHandler.prototype.resume = function() {
this.paused = false
}
Here's an interaction diagram explaining what I'm trying to achieve:
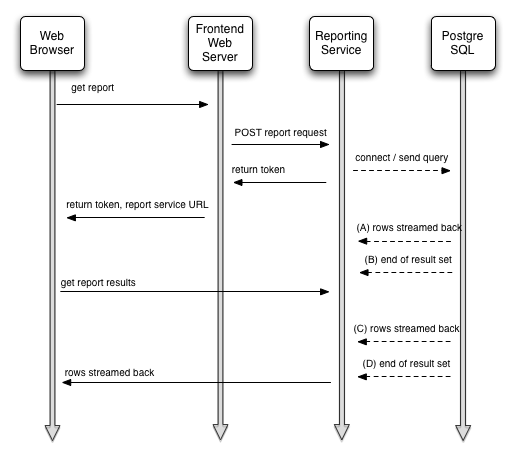
- The web browser asks the frontend webserver for a report
- The frontend webserver asks the reporting service for a token relating to a specific query
- Simultaneously, the reporting service connects to PostgreSQL and sends the query
- The frontend webserver returns the reporting service's URL and token back to the web browser
- The web browser does an Ajax (long polling) request to the reporting service with the returned token
- The reporting service streams rows as they come in back to the web browser
Because everything is asynchronous, step 3 might start returning data before the web browser connects, which would mean data loss. I could buffer the data in memory until the client comes back, but I'd rather block the emission of data rows, since that would prevent RAM use. Or maybe I'm just kidding myself and RAM will still be used in the underlying library? Anyway, pointers appreciated!
Source: (StackOverflow)